Developer Guide
- Acknowledgements
- Setting up, getting started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix: Requirements
- Appendix: Planned Enhancements
- Appendix: Instructions for manual testing
Acknowledgements
This project is based on the AddressBook-Level3 project created by the SE-EDU initiative.
Setting up, getting started
Refer to the guide Setting up and getting started.
Design

.puml
files used to create diagrams in this document docs/diagrams
folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
Main
(consisting of classes Main
and MainApp
) is in charge of the app launch and shut down.
- At app launch, it initializes the other components in the correct sequence, and connects them up with each other.
- At shut down, it shuts down the other components and invokes cleanup methods where necessary.
The bulk of the app’s work is done by the following four components:
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Commons
represents a collection of classes used by multiple other components.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysPerson
andAppointment
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
The sequence diagram below illustrates the interactions within the Logic
component, taking execute("delete 1")
API call as an example.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline continues till the end of diagram.
How the Logic
component works:
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,DeleteCommandParser
) and uses it to parse the command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,DeleteCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to delete a person).
Note that although this is shown as a single step in the diagram above (for simplicity), in the code it can take several interactions (between the command object and theModel
) to achieve. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
AddressBookParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddCommand
) which theAddressBookParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the address book data i.e., all
Person
objects andAppointment
objects (which are contained in aUniquePersonList
and aUniqueAppointmentList
object). - stores the currently ‘selected’
Person
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Person>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components).

Tag
list in the AddressBook
, which Person
references. This allows AddressBook
to only require one Tag
object per unique tag, instead of each Person
needing their own Tag
objects.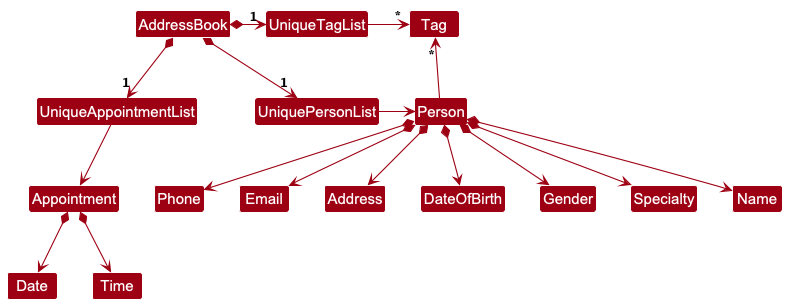
Storage component
API : Storage.java
The Storage
component,
- can save both address book data and user preference data in JSON format, and read them back into corresponding objects.
- inherits from both
AddressBookStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.address.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.
Add Patient and Add Doctor Features
This feature allows users to add new patients and doctors to MediContacts.
Given below is an example usage scenario and how the add-patient
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes add-patient
command, with all the required parameters and with valid inputs. The add-patient
command will indirectly call Model#addPerson()
to add the new patient to the address book.
See below sequence diagram for the add-patient
command:
The sequence of operations for add-doctor
is similar to add-patient
, with the only difference being that the add-doctor
command will indirectly call Model#addDoctor()
to add the new doctor to the address book.
Delete Patient and Delete Doctor Features
This feature allows users to delete patients and doctors from MediContacts.
Given below is an example usage scenario and how the delete
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes delete
command, with the index of the patient/doctor to be deleted. The delete
command will indirectly call Model#deletePerson()
to delete the patient/doctor from the address book.
See below sequence diagram for the delete
command:
List Patients and List Doctors Features
This feature allows users to list all patients and doctors stored in MediContacts.
Given below is an example usage scenario and how the list-patient
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes list-patient
command. The list-patient
command will indirectly call Model#getFilteredPersonList()
to get the list of patients/doctors to be displayed.
See below sequence diagram for the list
command:
The sequence of operations for list-doctor
is similar to list-patient
, with the only difference being that the list-doctor
command will indirectly call Model#getFilteredPersonList()
to get the list of doctors to be displayed.
Find Patient and Find Doctor Features
This feature allows users to find a specific patient or doctor stored in MediContacts.
Given below is an example usage scenario and how the find-patient
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes find-patient
command, with the name of the patient to be found. The find-patient
command will indirectly call Model#getFilteredPersonList()
to get the list of patients/doctors to be displayed.
Sequence Diagram is similar to the above list command, therefore not repeated here.
The sequence of operations for find-doctor
is similar to find-patient
, with the only difference being that the find-doctor
command will indirectly call Model#getFilteredPersonList()
to get the list of doctors to be displayed.
Add Appointment Feature
This feature allows users to add appointments for patients and doctors in MediContacts.
Given below is an example usage scenario and how the add-appt
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes add-appt
command, with all the required parameters and with valid inputs. The add-appt
command will indirectly call Model#addAppointment()
to add the new appointment to the address book.
See below sequence diagram for the add-appt
command:
Delete Appointment Feature
This feature allows users to delete appointments from MediContacts.
Given below is an example usage scenario and how the delete-appt
command behaves at each step.
Step 1. The user launches the application for the first time. MediContacts will be loaded in with the information stored in the addressbook.json
file.
Step 2. The user executes delete-appt
command, with the index of the appointment to be deleted. The delete-appt
command will indirectly call Model#deleteAppointment()
to delete the appointment from the address book.
See below sequence diagram for the delete-appt
command:
——————————————————————————————————————–
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile:
Our target user is a receptionist at a small clinic who:
- has a need to manage a significant number of patients and doctors contacts
- has a need to manage appointments for these contacts
- prefer desktop apps over other types
- can type fast
- prefers typing to mouse interactions
- values tools that allow for rapid data entry and retrieval
- requires robust search capabilities to quickly find patient or doctor information
Value proposition: streamlines clinic operations by organizing personnel records and simplifying appointment scheduling
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
receptionist | add new patient records | maintain an up-to-date list of patients |
* * * |
receptionist | delete outdated patient records | keep the patient database clean and relevant |
* * * |
receptionist | search for a patient’s record by their name | quickly retrieve their information |
* * * |
receptionist | add appointments for both patients and doctors | keep track of their respective appointments |
* * * |
receptionist | delete appointments for both patients and doctors | keep track of their respective appointments |
* * * |
user | list all the doctors stored in the address book. | see all doctors’ contact details in the address book |
* * * |
user | add doctor records | manage a list of all doctors working in the clinic |
* * * |
user | delete doctor records | manage a list of all doctors working in the clinic |
* * * |
user | find a specific doctor in the address book | check if a certain doctor’s details are stored in the address book |
* * |
receptionist | view a summary of all patient records | prepare for upcoming consultations |
* * |
receptionist | view upcoming appointments for each doctor | manage the clinic’s daily schedule effectively |
* * |
receptionist | tag certain patients according to their needs | search for patients based on their care requirements |
* * |
receptionist | tag certain patients according to their priorities | contact them when necessary |
* * |
receptionist* | update existing patient details | keep up with the latest information |
* * |
receptionist* | view which patients need to be called on the current date | so that I easily find out who to I need to contact them |
* * |
receptionist* | add reminders for certain days | keep track of all reminders/tasks for the given date |
* * |
receptionist* | update reminders I made | keep up with the latest information |
* * |
receptionist* | delete reminders I made | remove entries that have the wrong details. |
* * |
receptionist* | search for patients based on the tag given to them | easily find and identify patients |
* |
receptionist* | fetch the history of missed appointments | contact the patient and inform the doctor |
* * |
receptionist | search for patient based on their name/contact number | easily find their contact details and records |
* * |
receptionist* | generate a list of all upcoming appointments for the current date (or a specified one) | to assist in daily scheduling (which patient consult which doctor) and preparation |
* * |
receptionist* | filter patient records by the type of doctor/specialist they are meeting/have already consulted | manage referrals and specialist appointments effectively |
* * |
receptionist* | set recurring appointments for patients | streamline the process for those who require regular consultations (instead of them having to repeatedly schedule appointments) |
* * * |
receptionist | add/link a patient appointment to a specific doctor after checking the doctor’s availability | |
* * |
receptionist* | change/update a patient’s linked doctor if there is a sudden change in the doctor’s availability | |
* * |
user* | view records in a calendar view | get an organised overview of all appointments |
* |
user* | sort and view records based on their dates | |
* * |
user* | update the status of doctors (available, on leave, etc) | |
* * |
user* | track the availability of doctors and when they are free based on patient records | to help create appointments to patients |
* * |
healthcare provider* | add notes to patient records | |
* |
healthcare provider* | view a patient’s past appointments in this clinic | better understand their medical history and prepare their doctor for consultations |
* |
receptionist* | mark appointments done or undone | keep track of the appointments |
User stories tagged with * are considered as stretch goals and will be implemented in future versions of the product.
Use cases
(For all use cases below, the System is the MediContacts
and the Actor is the user
, unless specified otherwise)
Use case: UC01 - Add a patient
MSS
- User requests to add a patient
-
MediContacts adds the patient
Use case ends.
Extensions
-
1a. The given patient is a duplicate already in MediContacts.
-
1a1. MediContacts shows an error message.
Use case ends.
-
-
1b. The given name uses the wrong format.
-
1b1. MediContacts shows an error message.
Use case ends.
-
-
1c. The given phone number uses the wrong format.
-
1c1. MediContacts shows an error message.
Use case ends.
-
-
1d. The given email uses the wrong format.
-
1d1. MediContacts shows an error message.
Use case ends.
-
-
1e. The given address uses the wrong format.
-
1e1. MediContacts shows an error message.
Use case ends.
-
-
1f. The given date of birth uses the wrong format.
-
1f1. MediContacts shows an error message.
Use case ends.
-
-
1g. The given gender uses the wrong format.
-
1g1. MediContacts shows an error message.
Use case ends.
-
-
1h. The given tag(s) uses the wrong format.
-
1h1. MediContacts shows an error message.
Use case ends.
-
Use case: UC02 - Add a doctor
MSS
- User requests to add a doctor
-
MediContacts adds the doctor
Use case ends.
Extensions
-
1a. The given doctor is a duplicate already in the MediContacts.
-
1a1. MediContacts shows an error message.
Use case ends.
-
-
1b. The given name uses the wrong format.
-
1b1. MediContacts shows an error message.
Use case ends.
-
-
1c. The given phone number uses the wrong format.
-
1c1. MediContacts shows an error message.
Use case ends.
-
-
1d. The given email uses the wrong format.
-
1d1. MediContacts shows an error message.
Use case ends.
-
-
1e. The given address uses the wrong format.
-
1e1. MediContacts shows an error message.
Use case ends.
-
-
1f. The given speciality uses the wrong format.
-
1f1. MediContacts shows an error message.
Use case ends.
-
-
1g. The given tag(s) uses the wrong format.
-
1g1. MediContacts shows an error message.
Use case ends.
-
Use case: UC03 - List all patients
MSS
- User requests to list patients
-
MediContacts shows a list of all patients previously added
Use case ends.
Use case: UC04 - List all doctors
MSS
- User requests to list doctors
-
MediContacts shows a list of all doctors previously added
Use case ends.
Use case: UC05 - List all doctors and patients
MSS
- User requests to list all doctors and patients
-
MediContacts shows a list of all doctors and patients previously added
Use case ends.
Use case: UC06 - Find a patient
MSS
- User requests to find a specific patient
-
MediContacts shows a list of all patients with matching names
Use case ends.
Extensions
-
1a. The given name uses the wrong format.
-
1a1. MediContacts shows an error message.
Use case ends.
-
Use case: UC07 - Find a doctor
MSS
- User requests to find a specific doctor
-
MediContacts shows a list of all doctors with matching names
Use case ends.
Extensions
-
1a. The given name uses the wrong format.
-
1a1. MediContacts shows an error message.
Use case ends.
-
Use case: UC08 - Delete a patient
MSS
- User requests to either list patients (UC03) or find a patient (UC06)
- MediContacts shows a list of patients
- User requests to delete a specific patient in the list
-
MediContacts deletes the patient
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. MediContacts shows an error message.
Use case resumes at step 2.
-
Use case: UC09 - Delete a doctor
MSS
- User requests to either list doctors (UC04) or find a doctor (UC07)
- MediContacts shows a list of doctors
- User requests to delete a specific doctor in the list
-
MediContacts deletes the doctor
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. MediContacts shows an error message.
Use case resumes at step 2.
-
Use case: UC10 - Add an appointment
MSS
- User requests to add an appointment
-
MediContacts adds the appointment
Use case ends.
Extensions
-
1a. The given appointment is a duplicate already in the MediContacts.
-
1a1. MediContacts shows an error message.
Use case ends.
-
-
1b. The given appointment clashes with an existing appointment with the given doctor or patient.
-
1b1. MediContacts shows an error message.
Use case ends.
-
-
1b. The given patient name uses the wrong format.
-
1b1. MediContacts shows an error message.
Use case ends.
-
-
1c. The given doctor name uses the wrong format.
-
1c1. MediContacts shows an error message.
Use case ends.
-
-
1d. The given date uses the wrong format.
-
1d1. MediContacts shows an error message.
Use case ends.
-
-
1e. The given time uses the wrong format.
-
1e1. MediContacts shows an error message.
Use case ends.
-
Use case: UC11 - Delete an appointment
MSS
- User requests to delete an appointment with the given unique ID.
-
MediContacts deletes the appointment.
Use case ends.
Extensions
-
1a. The given unique ID is invalid.
- 3a1. MediContacts shows an error message.
Use case ends.
-
1b. The appointment with the given unique ID does not exist.
-
3b1. MediContacts shows an error message.
Use case ends.
{More to be added as more features get added}
Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
17
or above installed. - The system should be designed to allow the addition of new features, such as supporting other user types (e.g., nurses, staff) or integrating external systems, with minimal changes to the core codebase.
- The system must securely store patient and doctor information to comply with healthcare data privacy regulations, such as HIPAA.
- The system should log all user actions, such as adding, deleting, or modifying records. Logs should be stored for a minimum of 6 months and be accessible to authorized administrators for auditing purposes.
- The system should provide a response time of less than 2 seconds for any user interaction under normal load (i.e., up to 1000 patients and 500 doctors).
Glossary
- Mainstream OS: Windows, Linux, Unix, MacOS
- Healthcare Data Privacy Regulations: Laws and standards that govern the storage and access to patient and doctor data. Examples include HIPAA.
- HIPAA: The Health Insurance Portability and Accountability Act, a regulation in the U.S. that mandates secure handling of personal health information.
- Audit: A record of all changes made in the system, including who made the changes and when.
Appendix: Planned Enhancements
Team Size: 5
-
Update
add-patient
andadd-doctor
commands to check the date of birth and phone number input for logical inputs- Currently, both commands do not check whether the given data is a valid date of birth.
- We plan to make the commands check whether the data of birth given is not just in the correct format given, but also to check that it is:
- In the past (dates that are in the future from current date are illogical)
- Not too far in the past (e.g. 200 years ago from current date are illogical)
- We also plan to make the commands check whether the phone number given is not just in the correct format given, but also to check that it is:
- A valid phone number (e.g. 7 - 15 digits, handling the shortest and longest phone numbers internationally)
-
Make ‘successfully added patient/doctor’ message more detailed
- Both commands success message do not show all the details of the patient/doctor added. Specifically:
- The
add-patient
command does not show the patient’s date of birth and gender - The
add-doctor
command does not show the doctor’s speciality
- The
- We plan to make the success message show all the details of the patient/doctor added, including those above.
- Both commands success message do not show all the details of the patient/doctor added. Specifically:
-
Fixing issues with error messages.
- In the current implementation, some commands display the wrong error message when the user enters an invalid command.
- We plan to correct these instances:
-
delete-appt
should display “Invalid Unique ID, appointment does not exist.” when the index specified is too large, instead of “Invalid command format!”. (e.gdelete-appt 1000000000000000000000000
) -
delete
should display “The person index provided is invalid.” when index0
or a negative integer is entered. -
add-patient
should display “Invalid date of birth provided” when the date of birth is not a valid date (e.g 32-04-1995 does not exist), instead of the current generic message “Dates must be in the format of DD-MM-YYYY”. -
add-appt
should display “Invalid date provided” when the date is not a valid date (e.g 32-04-1995 does not exist), instead of the current generic message “Dates must be in the format of DD-MM-YYYY”. -
add-appt
should display “Invalid time provided” when the time is outside of the range 0000 - 2359, instead of the current generic message “Times must be in the format of HHmm”. - Giving invalid tags error messaage needs to be updated to let user know that tags should not only contain alphanumeric characters, but also not contain any spaces.
-
- Some messages also have the term “address book”. Though not entirely wrong, we plan to change this to “MediContacts” to maintain consistency with the application’s name.
-
Update
add-doctor
command to take in a wider range of specialties- Currently, the
add-doctor
command only allows for specialties with no spaces and only alphabets. - We plan to update the command to allow for specialties with spaces and some special characters (e.g.
Cardiovascular Surgeon
,General Practitioner
,Orthopedic Surgeon
) to be supported.
- Currently, the
-
Fix
find
command to behave similarly tofind-doctor
andfind-patient
- Currently,
find
command does not check for invalid input (e.gfind eth1/
), unlike ourfind-doctor
andfind-patient
commands. - We plan to update the
find
command to check for invalid input and display an error message if the input has an invalid characters (e.g special characters or numbers).
- Currently,
-
Implement Maximum Word Count for Fields
- There are no restrictions on the length of fields like name and address, which can lead to excessively long inputs that affect display and usability.
- We plan to implement a maximum character count for specific fields:
- Name field: Limit to 50 characters to ensure readability and prevent display issues.
- Address field: Limit to 100 characters, allowing detailed addresses without causing layout overflow.
- These restrictions will be enforced at both the command parsing level (to provide immediate feedback) and the model level (to ensure consistency).
- If the input exceeds the maximum length, the user will receive an error message explaining the character limit.
-
Increasing the maximum number of appointments.
- Currently, the maximum number of appointments that can be stored is 10,000.
- We plan to increase this limit to a number that cannot be realistically reached, while ensuring the appointment IDs are still concise.
-
Sort Appointment by Date
- Currently, the application displays appointments in an unsorted order, which may reduce the usability and effectiveness of the application. To enhance user experience, appointments will be automatically sorted by date. This enhancement will help receptionist view upcoming appointments more easily.
- Sorting of appointments will be in ascending order (earliest to latest).
-
Fix UI bug regarding date of birth
- Intended Behaviour: For months with less than 31 days, entering a date that is too large but below 32 will be automatically corrected. (e.g. 30-02-2024 will return 29-02-2024)
- Current Behaviour: Entering a date that is too large but below 32 will not be automatically corrected in the UI. (e.g. 30-02-2024 will return 30-02-2024). But, the date will be stored correctly in storage, hence restarting the app will show the corrected date.
- We plan to fix the UI to display the corrected date immediately after the user enters the date.
-
Add feature to update patient and doctor records
edit-patient
andedit-doctor
- Currently, there is no feature to update patient and doctor records. This feature will allow users to update patient and doctor records when there are changes in their details.
- The update feature will allow users to update patient and doctor details such as name, phone number, email, address, date of birth
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Adding a patient
-
Adding a patient
-
Test case:
add-patient n/John Doe p/98765432 e/johndoe@example.com a/123 Sengkang Drive 4 d/23-04-1950 g/M t/elderly
Expected: A new patient is added to the list. The status message shows the details of the added patient. Timestamp in the status bar is updated. -
Test case:
add-patient n/John Doe123 p/98765432 e/johndoe@example.com a/123 Sengkang Drive 4 d/23-04-1950 g/M t/elderly
Expected: Error message indicating the name format is wrong. No patient is added. Status bar remains the same.
-
Adding a doctor
-
Adding a doctor
-
Test case:
add-doctor n/Jane Doe p/91234567 e/janedoe@example.com a/456 Clementi Ave 3 s/Cardiology t/colleague
Expected: A new doctor is added to the list. The status message shows the details of the added doctor. Timestamp in the status bar is updated. -
Test case:
add-doctor n/Jane Doe123 p/91234567 e/janedoe@example.com a/456 Clementi Ave 3 s/Cardiology t/colleague
Expected: Error message indicating the name format is wrong. No doctor is added. Status bar remains the same.
-
Deleting a person
-
Deleting a person while all persons are being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No person is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
Expected: Similar to previous.
-
-
Deleting a person while a filtered list is being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. Filter the list using thefind
command. -
Test case:
delete 1
Expected: First contact in the filtered list is deleted. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No person is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
Expected: Similar to previous.
-
-
Deleting a person which has appointments linked to it
-
Prerequisites: Add an appointment for the person that is first in the list using the
add-appt
command. -
Test case:
delete 1
Expected: Error message indicating the person has appointments linked to it. No person is deleted.
-
Adding an appointment
-
Adding an appointment for a doctor and patient
-
Prerequisites: Ensure at least one doctor and one patient are added to the system. The patient or doctor to be deleted should not have any appointments.
-
Test case:
add-appt pn/John Doe dn/Jane Doe d/23-04-1987 t/1100
Expected: Appointment is successfully added for the doctor and patient. Confirmation message shows details of the appointment. -
Test case:
add-appt pn/John Doe dn/Jane Doe d/23-04-1987 t/1100
(Duplicate appointment)
Expected: Error message indicating the appointment already exists at the same date and time for the doctor and patient.
-
-
Adding an appointment with an invalid date or time
- Test case:
add-appt pn/John Doe dn/Jane Doe d/23-04-1987 t/
Expected: Error message indicating command format is wrong. No appointment is added.
- Test case:
Deleting an appointment
-
Deleting an appointment that exists
-
Prerequisites: Add an appointment for a doctor and patient using the
add-appt
command or appointment already exists. -
Test case:
delete-appt UNIQUE_ID
Expected: Appointment is successfully deleted. Confirmation message shows details of the deleted appointment. -
Test case:
delete-appt UNIQUE_ID
(Appointment already deleted)
Expected: Error message indicating the appointment does not exist.
-
-
Deleting an appointment using an invalid id.
- Test case:
delete-appt UNIQUE_ID
(UNIQUE_ID
is not a positive integer)
Expected: Error message indicating an invalid id. No appointment is deleted.
- Test case: